Introduction
The Function API allows developers to extend Shoplazza’s core business scenarios (such as cart price adjustments) by implementing custom logic. Developers must compile their business logic into a WebAssembly module, register it on the platform, and have it executed by the system when triggered.
For example, the Function API enables developers to insert custom logic into the cart or checkout process, modifying product prices or other relevant data. Developers can achieve these modifications by writing specific Functions tailored to their needs.
Integration Sequence
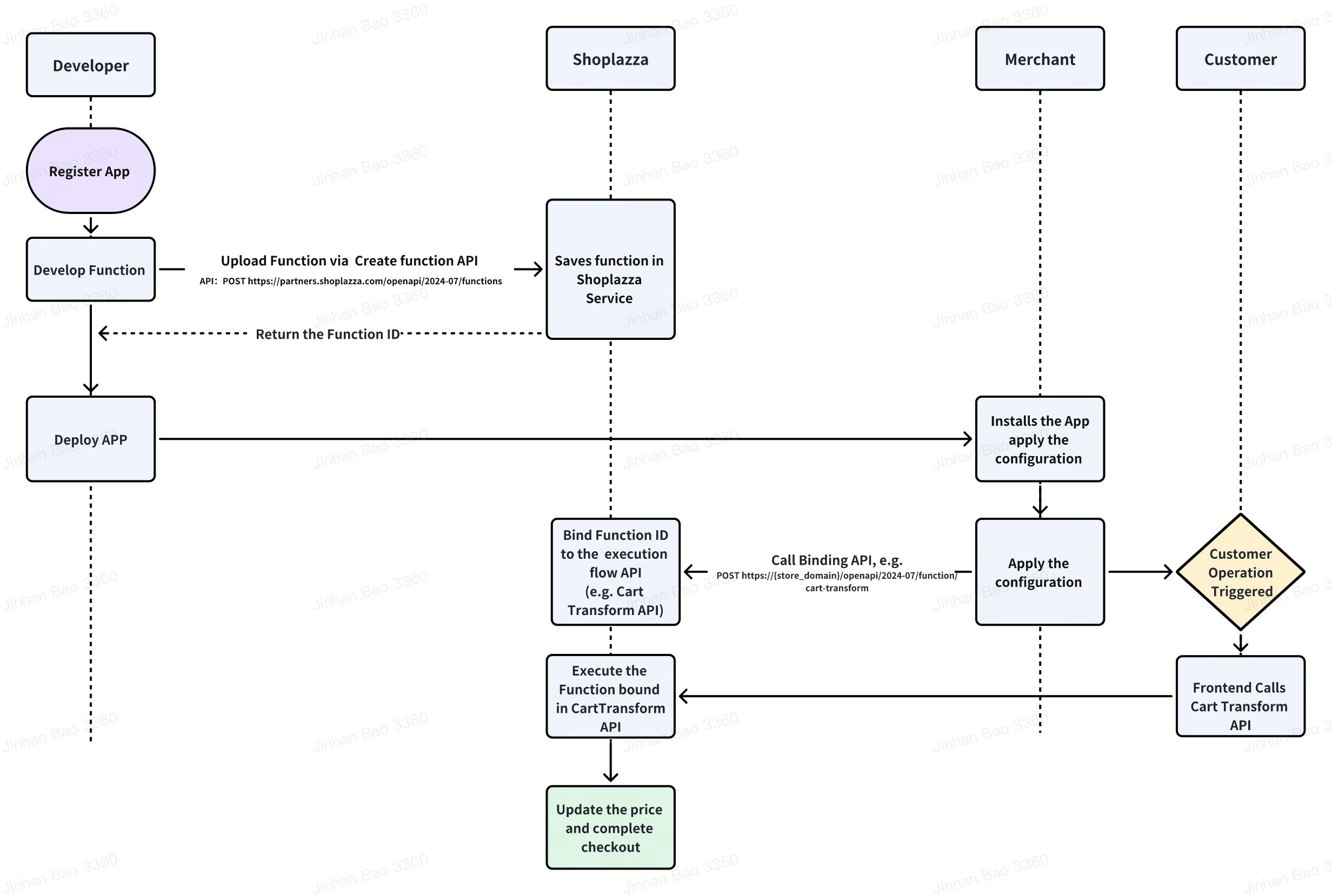
Function and Function API Implementation Guide
1. Understand Input and Output Protocols
- The Function receives JSON data from Shoplazza.
- The Function returns a set of operations used to adjust product prices.
2. Implement business logic of function
- Function scripts must be written in JavaScript or Rust.
- Limitations: Please note that the run function’s input and output size are constrained.
- function example:
function run(input) {
let runResult = new FunctionResult(new Operations([]));
input.cart.line_items.forEach(lineItem => {
lineItem.product.metafields.forEach(metafield => {
if (metafield.namespace === "custom-option" && metafield.key === "adjust-10-price") {
runResult.operations.update.push(new Update(
lineItem.id,
new PriceAdjustment(new AdjustmentPrice("10.00"))
));
}
});
});
return runResult;
}
3. Compile the Code into a WebAssembly File
- Compilation Toolchains:
- Rust: Use wasm-pack for building
- JavaScript: Use asc (AssemblyScript compiler) to generate WASM
- Output Requirement: .wasm file
- Limitations: The execution environment imposes restrictions on Wasm code. Please be aware of the following constraints during development:
- Fixed Limits: Wasm binary size, memory allocation, execution timeout, etc.
- Dynamic Limits: Maximum instruction execution count constraint
4. Update App Access Scopes
- Update the app configuration to enable relevant permissions, e.g., write_cart_transforms, read_cart_transforms.
Module | Read Permission | Write Permission | Permission Description |
---|---|---|---|
cart_transform | read_cart_transform | write_cart_transform | Read and modify cart and checkout transformation functions |
5. Upload Function Code to Shoplazza via the Create Function API
- Use the Create Function API to upload both the WASM file and the source code.
- Upon a successful upload, Shoplazza returns a function_id, which is required for registration and execution.
6. Register the Function to a Cart Pricing Adjustment Point
- When a third-party APP is installed in a merchant’s store, the merchant configures business rules (e.g., cart-transform)
- The APP Server registers the Function entry point on the Shoplazza platform using the functionID.
7. Execute Function in Business Processes
- When a customer adds items to the cart or proceeds to checkout, Shoplazza automatically executes the bound function.
- The function recalculates the price and updates the order accordingly, allowing users to view the adjusted prices in their shopping cart.
Authentication
Before calling the Shoplazza Open API, you need to complete OAuth 2.0 authentication. to obtain an Access Token. Follow the steps below to create an app and configure credentials:
Authentication Steps
1. Log in to your Shoplazza Partner Account and navigate to the Shoplazza Partner
2. In the left navigation bar, select “Apps”, then click the “Create App” button.
3. Enter the App Name and click Create. The system will automatically generate a Client ID and Client Secret, which can be found on the app settings page.
Obtain or Refresh Access Token
Function API requires an Access Token for requests. Use client_secret and client_id to obtain it.
Example Request (cURL)
curl --location 'https://partners.shoplazza.com/partner/oauth/token' \
--header 'Content-Type: application/json' \
--data '{
"client_id":"{app_client_id}",
"client_secret":"{app_client_secret}",
"grant_type":"client_credentials"
}'
Example Response (JSON)
{
"access_token":"YOUR ACCESS TOKEN",
"token_type":"Bearer",
"expires_in":31556951,
"created_at":1740793402,
"store_id":null,
"store_name":null,
"expires_at":1772350354,
"locale":"zh-CN"
}
Using the Access Token in Function API Requests
Access-Token: {access_token}
Available Logic Point APIs
Logic Point APIs | Description | Input Rules | Output Rules |
---|---|---|---|
Cart Transform Function | Bind a function to the cart price adjustment point. | cart: Cart object containing product details. | operation: Cart operation object containing price updates. |
Update Function: Update the function to the cart price adjustment point. | |||
Query Function: Query the current function details of the cart price adjustment point. | |||
Remove Function: Remove the function when no longer needed. |
Function Input and Output protocol
Function execution logic is fully defined by the developer, but must adhere to the structured input-output protocol. To ensure compatibility, the function must adhere to a strict input and output structure.
Function Execution Logic
When a function is registered at a specific execution point, Shoplazza automatically invokes it whenever the associated process occurs. The function receives structured input, processes it based on custom business rules, and returns an output that updates or modifies the relevant system data.
For a concrete example, see Cart-Transform Function, which modifies cart pricing dynamically.
Function Resource Limits
The following resource limits apply to:
Fixed Limits
The following resource limits apply to all functions:
Resource | Limit |
---|---|
Compiled binary size | 2048 kB |
Runtime linear memory | 10,000 kB |
Runtime stack memory | 512 kB |
Execution timeout (per function) | 300 ms |
Dynamic Limits
Certain limits are dynamic and apply when executing a function:
Resource | Limit |
---|---|
Maximum execution instructions | 11 million |
Function input data (run API) | ≤ 10 kB |
Function output data (run API) | ≤ 20 kB |